Welcome to Day 11 of 21 Days of AWS using Terraform, topic for today is Introduction to S3 using terraform.
What is AWS S3?
AWS Simple Storage Service(S3) provides secure, durable and highly scalable object storage. S3 is easy to use and we can store and retrieve any amount of data from anywhere on the web.
Let’s create S3 bucket using terraform
provider "aws" {
region = "us-west-2"
}
resource "aws_s3_bucket" "my-test-bucket" {
bucket = "${var.s3_bucket_name}"
acl = "private"
versioning {
enabled = true
}
tags = {
Name = "21-days-of-aws-using-terraform"
}
}
For more info
https://www.terraform.io/docs/providers/aws/r/s3_bucket.html
bucket: name of the bucket, if we ommit that terraform will assign random bucket name
acl: Default to Private(other options public-read and public-read-write)
versioning: Versioning automatically keeps up with different versions of the same object.
NOTE: Every S3 bucket must be unique and that why random id is useful to prevent our bucket to collide with others.
To implement that let use random_id as providers
resource "random_id" "my-random-id" {
byte_length = 2
}
byte_length
– The number of random bytes to produce. The minimum value is 1, which produces eight bits of randomness.
https://www.terraform.io/docs/providers/random/r/id.html
Now to use that in your code
provider "aws" {
region = "us-west-2"
}
resource "random_id" "my-random-id" {
byte_length = 2
}
resource "aws_s3_bucket" "my-test-bucket" {
bucket = "${var.s3_bucket_name}-${random_id.my-random-id.dec}"
acl = "private"
versioning {
enabled = true
}
tags = {
Name = "21-days-of-aws-using-terraform"
}
}
LifeCycle Management
Why do we need LifeCycle Management?
- To save cost
- In most of the cases, data which is generated by our application is relevant for us for the first 30 days and after that, we don’t access that data as frequently.
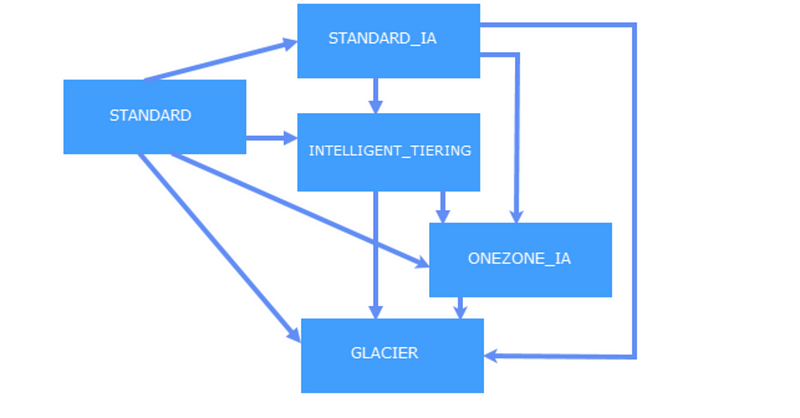
Reference:
https://docs.aws.amazon.com/AmazonS3/latest/dev/lifecycle-transition-general-considerations.html
LifeCycle object supports the following but I am going to enable just the required parameters
- enabled — (Required) Specifies lifecycle rule status.
transition
– (Optional) Specifies a period in the object’s transitions
resource "aws_s3_bucket" "my-test-bucket" {
bucket = "${var.s3_bucket_name}-${random_id.my-random-id.dec}"
acl = "private"
versioning {
enabled = true
}
lifecycle_rule {
enabled = true
transition {
storage_class = "STANDARD_IA"
days = 30
}
}
tags = {
Name = "21-days-of-aws-using-terraform"
}
}
- Here I am defining after 30 days move the objects to STANDARD_IA and after 60 days to GLACIER.
GitHub Link
https://github.com/100daysofdevops/21_days_of_aws_using_terraform/tree/master/s3
Looking forward for you guys to join this journey
- Website: https://100daysofdevops.com/
- Twitter: @100daysofdevops OR @lakhera2015
- Facebook: https://www.facebook.com/groups/795382630808645/
- Medium: https://medium.com/@devopslearning
- GitHub: https://github.com/100daysofdevops/100daysofdevops
- Slack: https://join.slack.com/t/100daysofdevops/shared_invite/enQtNzg1MjUzMzQzMzgxLWM4Yjk0ZWJiMjY4ZWE3ODBjZjgyYTllZmUxNzFkNTgxZjQ4NDlmZjkzODAwNDczOTYwOTM2MzlhZDNkM2FkMDA
- YouTube Channel: https://www.youtube.com/user/laprashant/videos?view_as=subscriber
In addition to that, I am going to host 5 meetups whose aim is to build the below architecture.

- Meetup: https://www.meetup.com/100daysofdevops
- Day1(Nov 10): Introduction to Terraform https://www.meetup.com/100daysofdevops/events/266192294/
- Day 2(Nov 16): Building VPC using Terraform
- Day 3(Nov 17): Creating EC2 Instance inside this VPC using Terraform
- Day 4(Nov 23): Adding Application Load Balancer and Auto-Scaling to the EC2 instance created on Day 3
- Day5(Nov 24): Add Backend MySQL Database and CloudWatch Alarm using Terraform